Optimizing performance in React applications is crucial for providing a seamless and responsive user experience. There are several strategies and best practices you can implement to achieve better performance. Here’s a guide on how to optimize your React application:
1. Profiler:
Example:
In the above example, after we started the profiler and entered the input, you can see the profiler shows how many times the component rendered and render is caused by which component.
2. React Memo:
Example:
Parent Component:
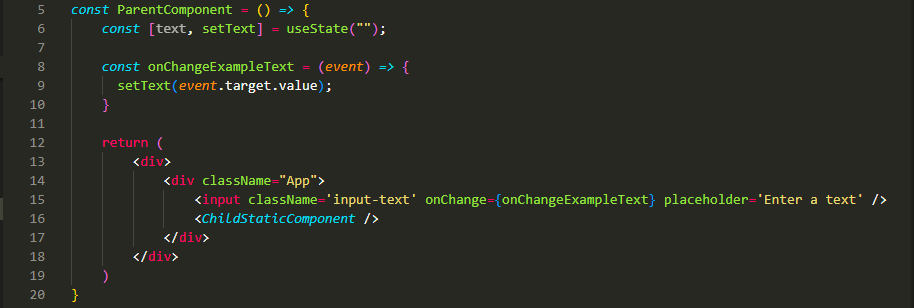
Child Component:
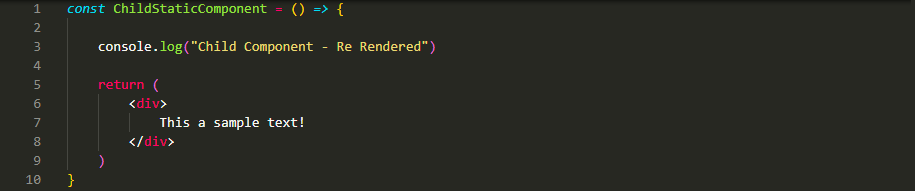
Without memo:
In the above example without memo the child component re renders every time when a user inputs a text, the child component re-renders.
Child Component - With Memo:
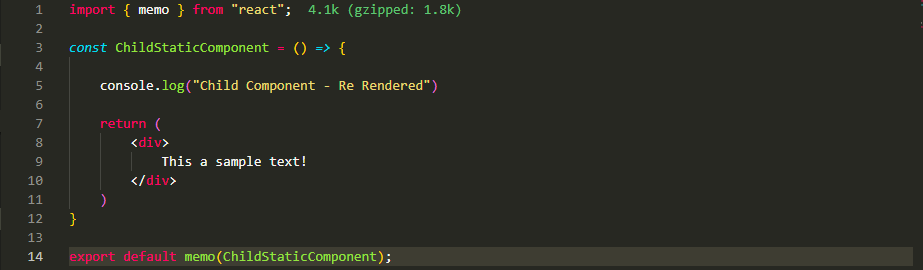
to prevent component from re rendering un necessarily, we add memo as HOC(higher order component) for child component, now child component does not re render when user enters a input.
3. Debounce and Throttle:
When dealing with user interactions or data updates, consider using debounce and throttle techniques to prevent excessive re-renders and API requests.
Debounce:
This is a function waits that delays invoking func until after wait have elapsed since the last time the debounced function was invoked
Example:
Parent Component:
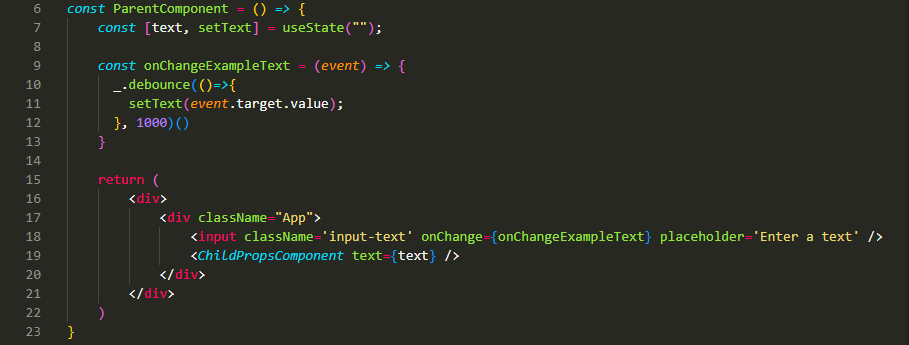
Child Component:
function ChildPropsComponent({ text }) {
console.log("Props Child Component - Re Rendered")
return (
This is a text from input: {text}!
)
}
Debounce:
In the above example, the debounce waits for 1 seconds after user stops inputting the text, and then it call the debounce function.
Throttle:
Throttling is a technique used to limit the number of times a function is executed over a specified time interval. It’s often used in React to optimize performance in scenarios like handling scroll or resize events to reduce excessive function calls. Here’s an example of how to implement throttle in a React component
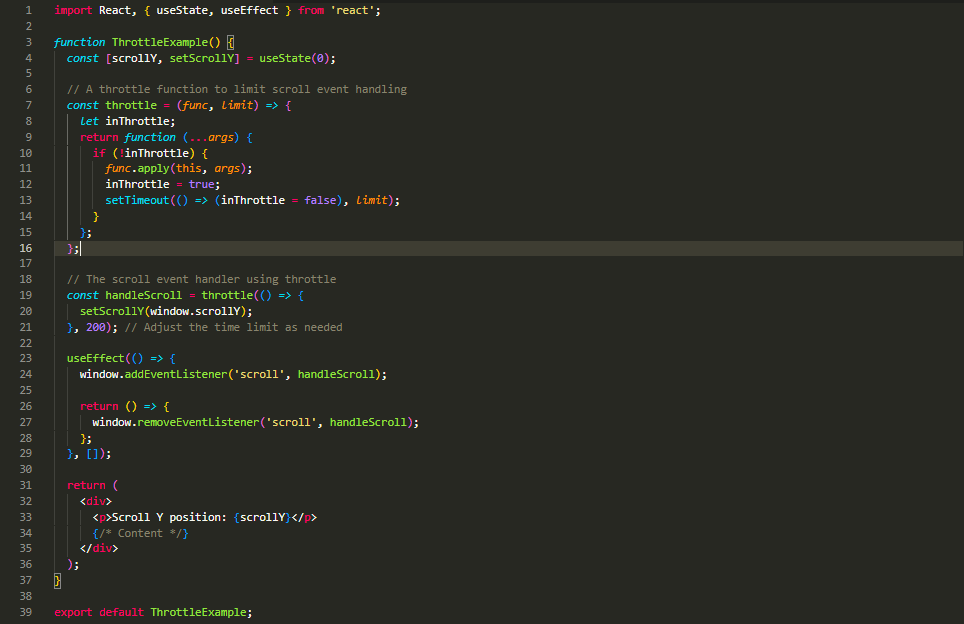
In this example, we create a throttle function that takes another function and a time limit as arguments. The throttle function returns a new function that wraps the original function. This new function will execute the original function at most once within the specified time limit.
In the useEffect, we add an event listener for the ‘scroll’ event that calls our throttled handleScroll function. When the component unmounts, we remove the event listener to avoid memory leaks. The scroll position is displayed in the component, but you can use the throttled function for other actions, like lazy loading images or triggering animations. Adjust the time limit in the throttle function according to your specific requirements.